Achieve Cert C Compliance Seamlessly with CppDepend
Introduction
In today's competitive software development landscape, maintaining high-quality and secure code is essential. The Cert C coding standard serves as a guideline for creating reliable and safe C applications. But how can developers efficiently achieve Cert C compliance in their projects?
Enter CppDepend, a comprehensive tool specifically designed to help you navigate the complexities of the Cert C standard. By utilizing static analysis techniques, CppDepend not only guides you towards compliance, but also empowers you to enhance overall code quality and security.
To learn Cert C Standards, you can print this SEI CERT C Coding Standard documentation by Carnegie Mellon University.
Using Cert C Rules in CppDepend
When you create a new CppDepend Project, a pop-up will appear displaying all the coding standards you would like to include in your analysis. To include Cert C coding standards, check the Cert option and choose Cert C.
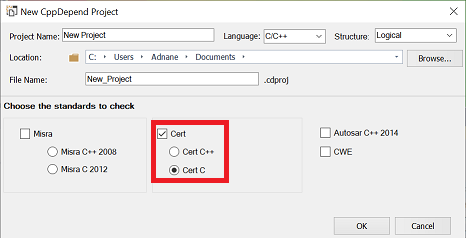
After analyzing your project, Navigate to the Queries and Rules Explorer section, and select the Cert C Rules from the left-hand menu (Highlighted in red in the image below). This will display all the related Cert C rules on the right. Upon clicking a Rule, the corresponding CQLinq query and the relevant source code will be automatically generated. Additionally, the Metrics View section provides a visualization of the issue's location and significance.
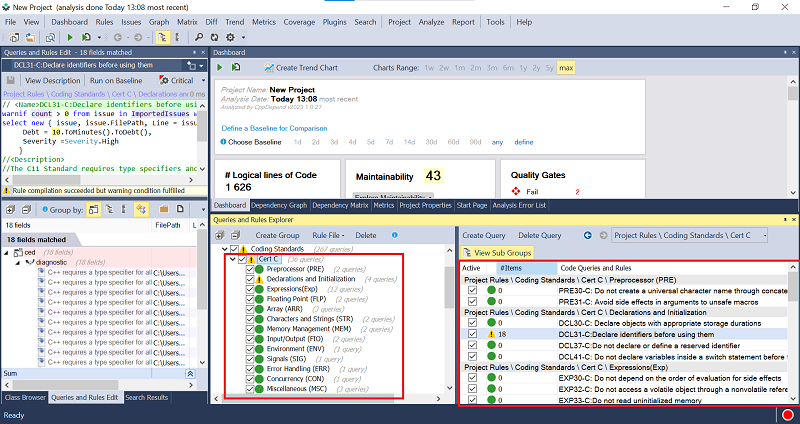
Incorporating Cert C Rules into the HTML Report
To include the Cert C rules in the generated HTML Report (available in the DevOps Edition), simply right-click on the Cert C group and select "List Code Queries of this Group in a dedicated section in Report."
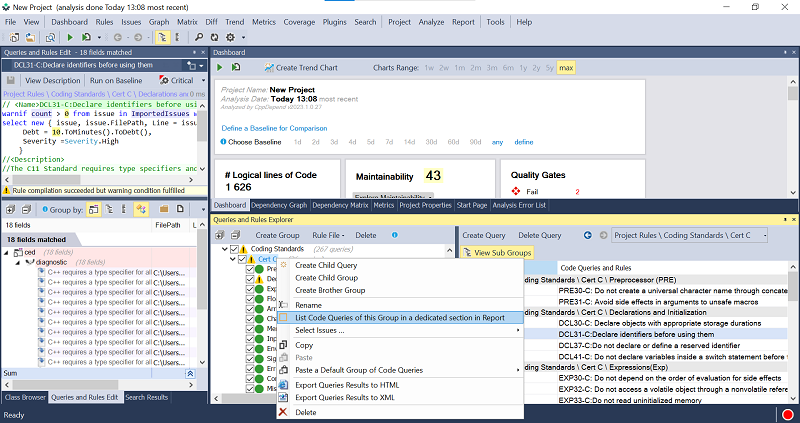
List of Cert C Rules
CppDepend offers support for 38 Cert C standard queries.To gain a deeper understanding of each rule and its functionality, please consult SEI CERT C Coding Standard documentation by Carnegie Mellon University.
- 2 Preprocessor (PRE),
- 4 Declarations and Initialization,
- 12 Expressions(Exp),
- 2 Floating Point (FLP),
- 3 Array (ARR),
- 2 Characters and Strings (STR),
- 2 Memory Management (MEM),
- 2 Input/Output (FIO),
- 1 Environment (ENV),
- 1 Signals (SIG),
- 1 Error Handling (ERR),
- 1 Concurrency (CON),
- 2 Miscellaneous (MSC).
Preprocessor (PRE)
-
PRE30-C: Do not create a universal character name through concatenation:
The C Standard supports universal character names that may be used in identifiers, character constants, and string literals to designate characters that are not in the basic character set. The universal character name \Unnnnnnnn designates the character whose 8-digit short identifier (as specified by ISO/IEC 10646) is nnnnnnnn. Similarly, the universal character name \unnnn designates
the character whose 4-digit short identifier is nnnn (and whose 8-digit short identifier is
0000nnnn).
The C Standard, 5.1.1.2, paragraph 4 [ISO/IEC 9899:2011], says
If a character sequence that matches the syntax of a universal character name is produced by token concatenation (6.10.3.3), the behavior is undefined.
In general, avoid universal character names in identifiers unless absolutely necessary.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_ucn_escape_incomplete"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
PRE31-C: Avoid side effects in arguments to unsafe macros:
An unsafe function-like macro is one whose expansion results in evaluating one of its parameters
more than once or not at all. Never invoke an unsafe macro with arguments containing an assignment, increment, decrement, volatile access, input/output, or other expressions with side effects
(including function calls, which may cause side effects).
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="bugprone-macro-repeated-side-effects"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}
Declarations and Initialization
-
DCL30-C: Declare objects with appropriate storage durations:
Every object has a storage duration that determines its lifetime: static, thread, automatic, or allocated.
According to the C Standard, 6.2.4, paragraph 2 [ISO/IEC 9899:2011],
The lifetime of an object is the portion of program execution during which storage is
guaranteed to be reserved for it. An object exists, has a constant address, and retains its
last-stored value throughout its lifetime. If an object is referred to outside of its lifetime,
the behavior is undefined. The value of a pointer becomes indeterminate when the object it points to reaches the end of its lifetime.
Do not attempt to access an object outside of its lifetime. Attempting to do so is undefined behavior and can lead to an exploitable vulnerability.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="danglingLifetime"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
DCL31-C:Declare identifiers before using them:
The C11 Standard requires type specifiers and forbids implicit function declarations. The C90
Standard allows implicit typing of variables and functions. Consequently, some existing legacy
code uses implicit typing. Some C compilers still support legacy code by allowing implicit typing,
but it should not be used for new code. Such an implementation may choose to assume an implicit
declaration and continue translation to support existing programs that used this feature.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="err_missing_type_specifier"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
DCL37-C:Do not declare or define a reserved identifier:
The behavior of a program that declares or defines an identifier in a context in
which it is reserved or that defines a reserved identifier as a macro name is undefined.
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_pp_macro_is_reserved_id" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
DCL41-C: Do not declare variables inside a switch statement before the first case label:
If a programmer declares variables, initializes them before the first case statement, and then tries
to use them inside any of the case statements, those variables will have scope inside the switch
block but will not be initialized and will consequently contain indeterminate values.
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.Type=="Rule6-4-4"
select new { issue,issue.FilePath,Line=issue.BeginLine}
Expressions(Exp)
-
EXP30-C: Do not depend on the order of evaluation for side effects:
Evaluation of an expression may produce side effects. At specific points during execution, known
as sequence points, all side effects of previous evaluations are complete, and no side effects of
subsequent evaluations have yet taken place. Do not depend on the order of evaluation for side effects unless there is an intervening sequence point.
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_unsequenced_mod_use" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP32-C: Do not access a volatile object through a nonvolatile reference:
An object that has volatile-qualified type may be modified in ways unknown to the implementation or have other unknown side effects. Referencing a volatile object by using a non-volatile
lvalue is undefined behavior. The C Standard, 6.7.3 [ISO/IEC 9899:2011], states
If an attempt is made to refer to an object defined with a volatile-qualified type through
use of an lvalue with non-volatile-qualified type, the behavior is undefined.
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang"
&& issue.Type=="err_typecheck_convert_incompatible_volatile" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP33-C:Do not read uninitialized memory:
Local, automatic variables assume unexpected values if they are read before they are initialized.
The C Standard, 6.7.9, paragraph 10, specifies [ISO/IEC 9899:2011]
If an object that has automatic storage duration is not initialized explicitly, its value is indeterminate.
When local, automatic variables are stored on the program stack, for example, their values default
to whichever values are currently stored in stack memory.
Additionally, some dynamic memory allocation functions do not initialize the contents of the
memory they allocate.
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="CppCheck" && (issue.Type=="uninitvar" || issue.Type=="uninitdata") select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP34-C: Do not dereference null pointers:
Dereferencing a null pointer is undefined behavior.
On many platforms, dereferencing a null pointer results in abnormal program termination, but this
is not required by the standard.
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="nullPointer" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP35-C: Do not modify objects with temporary lifetime:
The C11 Standard [ISO/IEC 9899:2011] introduced a new term: temporary lifetime. Modifying an
object with temporary lifetime is undefined behavior. According to subclause 6.2.4, paragraph 8
A non-lvalue expression with structure or union type, where the structure or union contains a member with array type (including, recursively, members of all contained structures and unions) refers to an object with automatic storage duration and temporary lifetime. Its lifetime begins when the expression is evaluated and its initial value is the value
of the expression. Its lifetime ends when the evaluation of the containing full expression
or full declarator ends. Any attempt to modify an object with temporary lifetime results in
undefined behavior.
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang"
&& issue.Type=="err_typecheck_expression_not_modifiable_lvalue" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP36-C: Do not cast pointers into more strictly aligned pointer types:
Do not convert a pointer value to a pointer type that is more strictly aligned than the referenced
type. Different alignments are possible for different types of objects. If the type-checking system
is overridden by an explicit cast or the pointer is converted to a void pointer (void *) and then to
a different type, the alignment of an object may be changed.
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_cast_align" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP37-C: Call functions with the correct number and type of arguments:
Do not call a function with the wrong number or type of arguments.
The C Standard identifies five distinct situations in which undefined behavior (UB) may arise as a
result of invoking a function using a declaration that is incompatible with its definition or by supplying incorrect types or numbers of arguments.
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="err_typecheck_call_too_many_args" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP39-C: Do not access a variable through a pointer of an incompatible type:
Modifying a variable through a pointer of an incompatible type (other than unsigned char) can
lead to unpredictable results. Subclause 6.2.7 of the C Standard states that two types may be distinct yet compatible and addresses precisely when two distinct types are compatible.
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="invalidPointerCast" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP40-C: Do not modify constant objects:
The C Standard, 6.7.3, paragraph 6 [ISO/IEC 9899:2011], states
If an attempt is made to modify an object defined with a const-qualified type through
use of an lvalue with non-const-qualified type, the behavior is undefined.
See also undefined behavior 64.
There are existing compiler implementations that allow const-qualified objects to be modified
without generating a warning message.
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="err_typecheck_convert_incompatible_const" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP44-C: Do not rely on side effects in operands to sizeof,_Alignof, or _Generic:
Some operators do not evaluate their operands beyond the type information the operands provide.
When using one of these operators, do not pass an operand that would otherwise yield a side effect since the side effect will not be generated.
The sizeof operator yields the size (in bytes) of its operand, which may be an expression or the
parenthesized name of a type. In most cases, the operand is not evaluated. A possible exception
is when the type of the operand is a variable length array type (VLA); then the expression is evaluated. When part of the operand of the sizeof operator is a VLA type and when changing the
value of the VLA’s size expression would not affect the result of the operator, it is unspecified
whether or not the size expression is evaluated.
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_side_effects_unevaluated_context"
&& issue.Description.Contains(("lambda")) select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP45-C:Do not perform assignments in selection statements:
Do not use the assignment operator in the contexts listed in the following table because doing so
typically indicates programmer error and can result in unexpected behavior.
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_condition_is_assignment" select
new { issue,issue.FilePath,Line=issue.BeginLine} -
EXP46-C. Do not use a bitwise operator with a Boolean-like operand:
Mixing bitwise and relational operators in the same full expression can be a sign of a logic error
in the expression where a logical operator is usually the intended operator. Do not use the bitwise
AND (&), bitwise OR (|), or bitwise XOR (^) operators with an operand of type _Bool, or the result of a relational-expression or equality-expression. If the bitwise operator is intended, it should
be indicated with use of a parenthesized expression.
This coding standard is computed with the following query:
warnif count > 0
from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_precedence_bitwise_rel" select
new { issue,issue.FilePath,Line=issue.BeginLine}
Floating Point (FLP)
-
FLP30-C:Do not use floating-point variables as loop counters:
Because floating-point numbers represent real numbers, it is often mistakenly assumed that they
can represent any simple fraction exactly. Floating-point numbers are subject to representational
limitations just as integers are, and binary floating-point numbers cannot represent all real numbers exactly, even if they can be represented in a small number of decimal digits.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="cert-flp30-c"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
FLP34-C: Ensure that floating-point conversions are within range of the new type:
If a floating-point value is to be converted to a floating-point value of a smaller range and precision or to an integer type, or if an integer type is to be converted to a floating-point type, the value
must be representable in the destination type.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_impcast_float_integer"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}
Array (ARR)
-
ARR30-C. Do not form or use out-of-bounds pointers or array subscripts:
Addition or subtraction of a pointer
into, or just beyond, an array object and an integer type produces
a result that does not point into, or
just beyond, the same array object.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="arrayIndexOutOfBounds"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
ARR36-C: Do not subtract or compare two pointers that do not refer to the same array:
When two pointers are subtracted, both must point to elements of the same array object or just one
past the last element of the array object (C Standard, 6.5.6 [ISO/IEC 9899:2011]); the result is the
difference of the subscripts of the two array elements. Otherwise, the operation is undefined behavior.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="comparePointers"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
ARR39-C: Do not add or subtract a scaled integer to a pointer:
Pointer arithmetic is appropriate only when the pointer argument refers to an array, including an
array of bytes. (See ARR37-C. Do not add or subtract an integer to a pointer to a non-array object.) When performing pointer arithmetic, the size of the value to add to or subtract from a
pointer is automatically scaled to the size of the type of the referenced array object. Adding or
subtracting a scaled integer value to or from a pointer is invalid because it may yield a pointer that
does not point to an element within or one past the end of the array.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_ptr_arith_exceeds_bounds"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}
Characters and Strings (STR)
-
STR30-C: Do not attempt to modify string literals:
At compile time, string literals are used to create an array of static storage duration of sufficient
length to contain the character sequence and a terminating null character. String literals are usually referred to by a pointer to (or array of) characters. Ideally, they should be assigned only to
pointers to (or arrays of) const char or const wchar_t. It is unspecified whether these arrays
of string literals are distinct from each other. The behavior is undefined if a program attempts to
modify any portion of a string literal. Modifying a string literal frequently results in an access violation because string literals are typically stored in read-only memory.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="stringLiteralWrite"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
STR34-C: Cast characters to unsigned char before converting to larger integer sizes:
Signed character data must be converted to unsigned char before being assigned or converted
to a larger signed type. This rule applies to both signed char and (plain) char characters on
implementations where char is defined to have the same range, representation, and behaviors as
signed char.
However, this rule is applicable only in cases where the character data may contain values that
can be interpreted as negative numbers. For example, if the char type is represented by a two’s
complement 8-bit value, any character value greater than +127 is interpreted as a negative value.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="bugprone-signed-char-misuse"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}
Memory Management (MEM)
-
MEM30-C:Do not access freed memory:
Evaluating a pointer—including dereferencing the pointer, using it as an operand of an arithmetic
operation, type casting it, and using it as the right-hand side of an assignment—into memory that
has been deallocated by a memory management function is undefined behavior. Pointers to
memory that has been deallocated are called dangling pointers. Accessing a dangling pointer can
result in exploitable vulnerabilities.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="nullPointer"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
MEM31-C. Free dynamically allocated memory when no longer needed:
Before the lifetime of the last pointer that stores the return value of a call to a standard memory
allocation function has ended, it must be matched by a call to free() with that pointer value.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang" && issue.Type=="warn_mismatched_delete_new"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}
Input/Output (FIO)
-
FIO38-C: Do not copy a FILE object:
According to the C Standard, 7.21.3, paragraph 6 [ISO/IEC 9899:2011],
The address of the FILE object used to control a stream may be significant; a copy of a
FILE object need not serve in place of the original.
Consequently, do not copy a FILE object.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="cert-fio38-c"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
FIO42-C: Close files when they are no longer needed:
A call to the fopen() or freopen() function must be matched with a call to fclose() before
the lifetime of the last pointer that stores the return value of the call has ended or before normal
program termination, whichever occurs first.
In general, this rule should also be applied to other functions with open and close resources, such
as the POSIX open() and close() functions, or the Microsoft Windows CreateFile() and
CloseHandle() functions.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="resourceLeak"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}
Environment (ENV)
-
ENV33-C: Do not call system():
The C Standard system() function executes a specified command by invoking an implementation-defined command processor, such as a UNIX shell or CMD.EXE in Microsoft Windows. The
POSIX popen() and Windows _popen() functions also invoke a command processor but create a
pipe between the calling program and the executed command, returning a pointer to a stream that
can be used to either read from or write to the pipe [IEEE Std 1003.1:2013].
Use of the system() function can result in exploitable vulnerabilities, in the worst case allowing
execution of arbitrary system commands.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="CppCheck" && issue.Type=="nullPointer"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}
Signals (SIG)
-
SIG30-C: Call only asynchronous-safe functions within signal handlers:
Call only asynchronous-safe functions within signal handlers. For strictly conforming programs,
only the C standard library functions abort(), _Exit(), quick_exit(), and signal() can be
safely called from within a signal handler.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="bugprone-signal-handler"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}
Error Handling (ERR)
-
ERR34-C: Detect errors when converting a string to a number:
The cnd_wait() and cnd_timedwait() functions temporarily cede possession of a mutex so
that other threads that may be requesting the mutex can proceed. These functions must always be
called from code that is protected by locking a mutex. The waiting thread resumes execution only
after it has been notified, generally as the result of the invocation of the cnd_signal() or
cnd_broadcast() function invoked by another thread. The cnd_wait() function must be invoked from a loop that checks whether a condition predicate holds. A condition predicate is an expression constructed from the variables of a function that must be true for a thread to be allowed
to continue execution. The thread pauses execution, via cnd_wait(), cnd_timedwait(), or
some other mechanism, and is resumed later, presumably when the condition predicate is true and
the thread is notified.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="cert-err34-c"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}
Concurrency (CON)
-
CON36-C. Wrap functions that can spuriously wake up in a loop:
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="bugprone-spuriously-wake-up-functions"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}
Miscellaneous (MSC)
-
MSC30-C: Do not use the rand() function for generating pseudorandom numbers:
Pseudorandom number generators use mathematical algorithms to produce a sequence of numbers
with good statistical properties, but the numbers produced are not genuinely random.
The C Standard rand() function makes no guarantees as to the quality of the random sequence
produced. The numbers generated by some implementations of rand() have a comparatively
short cycle and the numbers can be predictable. Applications that have strong pseudorandom
number requirements must use a generator that is known to be sufficient for their needs.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="cert-msc30-c"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
} -
MSC32-C: Properly seed pseudorandom number generators:
A pseudorandom number generator (PRNG) is a deterministic algorithm capable of generating sequences of numbers that approximate the properties of random numbers. Each sequence is completely determined by the initial state of the PRNG and the algorithm for changing the state. Most
PRNGs make it possible to set the initial state, also called the seed state. Setting the initial state is
called seeding the PRNG.
Calling a PRNG in the same initial state, either without seeding it explicitly or by seeding it with
the same value, results in generating the same sequence of random numbers in different runs of
the program. Consider a PRNG function that is seeded with some initial seed value and is consecutively called to produce a sequence of random numbers, S. If the PRNG is subsequently seeded
with the same initial seed value, then it will generate the same sequence S.
This coding standard is computed with the following query:
warnif count > 0 from issue in ImportedIssues where issue.ToolName=="Clang-Tidy" && issue.Type=="cert-msc32-c"
select new { issue, issue.FilePath, Line = issue.BeginLine ,
Debt = 10.ToMinutes().ToDebt(),
Severity =Severity.High
}